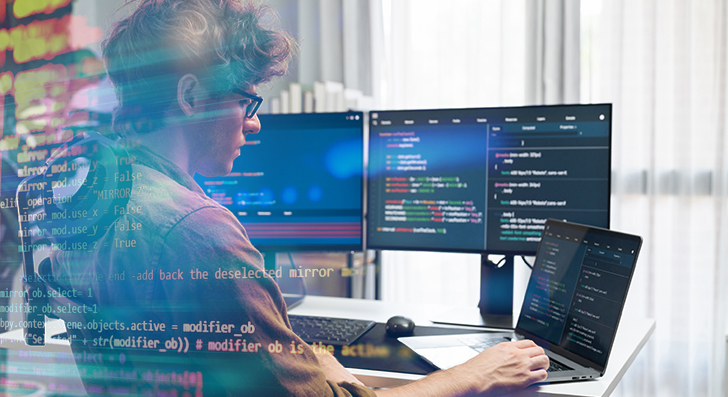
Scalability implies your software can cope with progress—much more users, extra knowledge, and a lot more traffic—devoid of breaking. Being a developer, developing with scalability in your mind saves time and worry later on. Right here’s a transparent and realistic guidebook that will help you get started by Gustavo Woltmann.
Layout for Scalability from the Start
Scalability isn't really some thing you bolt on afterwards—it should be part of your respective strategy from the start. Numerous apps fail whenever they develop rapid simply because the original layout can’t handle the extra load. To be a developer, you should Assume early about how your technique will behave stressed.
Start out by designing your architecture to get adaptable. Steer clear of monolithic codebases wherever everything is tightly linked. As a substitute, use modular style or microservices. These designs break your app into more compact, unbiased parts. Each and every module or assistance can scale By itself with out impacting The full method.
Also, think of your databases from working day a person. Will it require to deal with 1,000,000 people or simply just a hundred? Select the suitable style—relational or NoSQL—based on how your information will expand. Prepare for sharding, indexing, and backups early, Even though you don’t will need them nonetheless.
Yet another critical place is to stop hardcoding assumptions. Don’t generate code that only is effective under current circumstances. Consider what would take place Should your person foundation doubled tomorrow. Would your application crash? Would the database slow down?
Use style patterns that assistance scaling, like message queues or event-pushed methods. These assist your app handle more requests without having overloaded.
Whenever you Construct with scalability in your mind, you are not just getting ready for success—you're reducing future problems. A perfectly-prepared program is easier to maintain, adapt, and develop. It’s much better to prepare early than to rebuild afterwards.
Use the ideal Databases
Selecting the correct database is a vital A part of building scalable purposes. Not all databases are created the identical, and using the Completely wrong one can slow you down or maybe result in failures as your app grows.
Start by understanding your facts. Could it be highly structured, like rows in a very table? If Certainly, a relational database like PostgreSQL or MySQL is a good in good shape. These are typically potent with associations, transactions, and regularity. They also aid scaling tactics like read replicas, indexing, and partitioning to manage more website traffic and information.
If the facts is more versatile—like person activity logs, product or service catalogs, or documents—look at a NoSQL option like MongoDB, Cassandra, or DynamoDB. NoSQL databases are greater at managing huge volumes of unstructured or semi-structured data and might scale horizontally extra very easily.
Also, look at your read and publish styles. Are you currently undertaking many reads with fewer writes? Use caching and skim replicas. Are you currently dealing with a significant write load? Consider databases that could tackle higher compose throughput, or maybe party-based facts storage units like Apache Kafka (for temporary info streams).
It’s also sensible to Assume in advance. You might not need Sophisticated scaling functions now, but selecting a databases that supports them usually means you won’t need to switch later.
Use indexing to speed up queries. Steer clear of needless joins. Normalize or denormalize your info dependant upon your entry designs. And normally observe databases general performance when you mature.
To put it briefly, the ideal databases relies on your application’s framework, pace requires, And exactly how you be expecting it to improve. Acquire time to choose properly—it’ll conserve lots of trouble afterwards.
Improve Code and Queries
Rapidly code is vital to scalability. As your app grows, each and every little hold off provides up. Badly composed code or unoptimized queries can slow down general performance and overload your process. That’s why it’s essential to Create effective logic from the start.
Begin by crafting cleanse, basic code. Steer clear of repeating logic and take away nearly anything needless. Don’t choose the most elaborate Resolution if a simple a person is effective. Maintain your functions small, targeted, and straightforward to check. Use profiling tools to uncover bottlenecks—areas where your code can take also extensive to operate or employs too much memory.
Upcoming, take a look at your databases queries. These frequently gradual issues down much more than the code by itself. Be certain Each and every question only asks for the information you truly want. Stay clear of Pick *, which fetches all the things, and as an alternative find certain fields. Use indexes to hurry up lookups. And avoid undertaking a lot of joins, Particularly throughout big tables.
When you notice precisely the same details becoming asked for many times, use caching. Shop the outcome quickly using resources like Redis or Memcached therefore you don’t need to repeat high-priced functions.
Also, batch your databases operations whenever you can. As an alternative to updating a row one after the other, update them in teams. This cuts down on overhead and makes your app a lot more productive.
Make sure to exam with huge datasets. Code and queries that operate high-quality with a hundred documents might crash once they have to deal with 1 million.
In a nutshell, scalable apps are rapidly applications. Maintain your code restricted, your queries lean, and use caching when essential. These steps assist your application stay smooth and responsive, even as the load increases.
Leverage Load Balancing and Caching
As your app grows, it has to take care of more users and more visitors. If every thing goes by way of just one server, it can speedily turn into a bottleneck. That’s in which load balancing and caching are available in. These two tools assistance keep the application rapidly, stable, and scalable.
Load balancing spreads incoming visitors across numerous servers. Rather than one server doing many of the get the job done, the load balancer routes end users to distinct servers according to availability. This means no one server will get overloaded. If 1 server goes down, the load balancer can send visitors to the Other individuals. here Tools like Nginx, HAProxy, or cloud-primarily based solutions from AWS and Google Cloud make this straightforward to put in place.
Caching is about storing information quickly so it could be reused rapidly. When buyers ask for exactly the same information and facts once again—like a product site or even a profile—you don’t need to fetch it from your databases whenever. You are able to provide it in the cache.
There's two frequent different types of caching:
1. Server-facet caching (like Redis or Memcached) retailers details in memory for rapidly access.
2. Shopper-aspect caching (like browser caching or CDN caching) shops static documents close to the consumer.
Caching cuts down database load, increases speed, and can make your application a lot more economical.
Use caching for things that don’t transform frequently. And constantly ensure your cache is current when information does adjust.
In short, load balancing and caching are straightforward but impressive resources. Jointly, they assist your app manage additional users, remain rapid, and Get better from issues. If you intend to grow, you will need both equally.
Use Cloud and Container Tools
To construct scalable programs, you require applications that let your app expand simply. That’s where by cloud platforms and containers come in. They give you flexibility, minimize setup time, and make scaling much smoother.
Cloud platforms like Amazon Internet Providers (AWS), Google Cloud Platform (GCP), and Microsoft Azure Allow you to lease servers and companies as you require them. You don’t really have to buy components or guess future capacity. When visitors raises, you are able to include a lot more assets with just some clicks or quickly applying vehicle-scaling. When website traffic drops, you could scale down to economize.
These platforms also present expert services like managed databases, storage, load balancing, and protection equipment. It is possible to give attention to creating your app instead of running infrastructure.
Containers are A further critical Device. A container offers your app and every little thing it must operate—code, libraries, options—into a single unit. This can make it uncomplicated to move your app involving environments, from the laptop into the cloud, devoid of surprises. Docker is the most well-liked tool for this.
Once your app makes use of numerous containers, applications like Kubernetes allow you to control them. Kubernetes handles deployment, scaling, and Restoration. If one particular component of your application crashes, it restarts it immediately.
Containers also enable it to be simple to separate portions of your app into expert services. You'll be able to update or scale pieces independently, that's great for functionality and dependability.
In short, employing cloud and container tools suggests you are able to scale rapid, deploy effortlessly, and Get well quickly when challenges happen. In order for you your application to expand without the need of limitations, start out using these equipment early. They help you save time, decrease possibility, and enable you to continue to be focused on creating, not correcting.
Monitor Every little thing
When you don’t monitor your application, you gained’t know when points go wrong. Monitoring will help the thing is how your application is performing, spot troubles early, and make superior decisions as your app grows. It’s a important Element of building scalable techniques.
Start off by monitoring essential metrics like CPU use, memory, disk space, and response time. These tell you how your servers and providers are undertaking. Instruments like Prometheus, Grafana, Datadog, or New Relic will let you collect and visualize this data.
Don’t just keep track of your servers—check your app also. Keep watch over just how long it requires for end users to load web pages, how frequently faults materialize, and where they occur. Logging tools like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly can help you see what’s going on within your code.
Build alerts for significant challenges. One example is, If the reaction time goes previously mentioned a limit or perhaps a services goes down, you should get notified immediately. This allows you take care of difficulties rapidly, typically just before end users even recognize.
Monitoring is usually handy any time you make adjustments. In the event you deploy a new aspect and find out a spike in mistakes or slowdowns, you can roll it again just before it leads to serious problems.
As your app grows, traffic and facts boost. Without checking, you’ll skip indications of problems till it’s as well late. But with the ideal equipment set up, you keep on top of things.
In a nutshell, monitoring will help you keep your application reliable and scalable. It’s not almost spotting failures—it’s about comprehension your method and making certain it works properly, even under pressure.
Ultimate Views
Scalability isn’t just for major businesses. Even smaller apps need to have a strong foundation. By coming up with cautiously, optimizing wisely, and using the suitable tools, it is possible to build apps that improve smoothly with no breaking stressed. Start modest, Imagine large, and Create good.